mirror of
https://github.com/actions/setup-python.git
synced 2024-09-19 22:30:04 +07:00
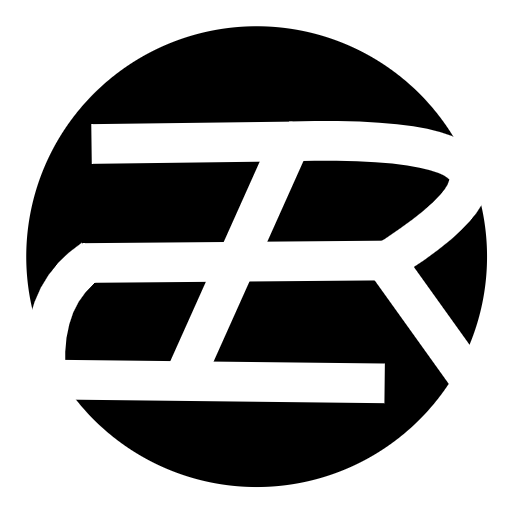
This pull-request improves `setup-python` action to add ability to download specific version of Python on flight if it is not available by default. **Details:** `setup-python` action will download and install specific Python version from GitHub releases ([actions/python-versions](https://github.com/actions/python-versions/releases)) in case the version is not found in the local cache. All versions of Python available for installation are published in [actions/python-versions](https://github.com/actions/python-versions) repository. All available versions are listed in the [version-manifest.json](https://github.com/actions/python-versions/blob/master/versions-manifest.json) file. **Installation time:** - Ubuntu / macOS: 10-20 seconds - Windows: ~ 1 minute (mostly related to fact that we use MSI installer for Python on Windows) Co-authored-by: MaksimZhukov <v-mazhuk@microsoft.com> Co-authored-by: Konrad Pabjan <konradpabjan@github.com> Co-authored-by: Brian Cristante <33549821+brcrista@users.noreply.github.com>
80 lines
2.5 KiB
TypeScript
80 lines
2.5 KiB
TypeScript
import io = require('@actions/io');
|
|
import fs = require('fs');
|
|
import path = require('path');
|
|
|
|
const toolDir = path.join(
|
|
__dirname,
|
|
'runner',
|
|
path.join(Math.random().toString(36).substring(7)),
|
|
'tools'
|
|
);
|
|
const tempDir = path.join(
|
|
__dirname,
|
|
'runner',
|
|
path.join(Math.random().toString(36).substring(7)),
|
|
'temp'
|
|
);
|
|
|
|
process.env['RUNNER_TOOL_CACHE'] = toolDir;
|
|
process.env['RUNNER_TEMP'] = tempDir;
|
|
|
|
import * as tc from '@actions/tool-cache';
|
|
import * as finder from '../src/find-python';
|
|
import * as installer from '../src/install-python';
|
|
|
|
const pythonRelease = require('./data/python-release.json');
|
|
|
|
describe('Finder tests', () => {
|
|
afterEach(() => {
|
|
jest.resetAllMocks();
|
|
jest.clearAllMocks();
|
|
});
|
|
|
|
it('Finds Python if it is installed', async () => {
|
|
const pythonDir: string = path.join(toolDir, 'Python', '3.0.0', 'x64');
|
|
await io.mkdirP(pythonDir);
|
|
fs.writeFileSync(`${pythonDir}.complete`, 'hello');
|
|
// This will throw if it doesn't find it in the cache and in the manifest (because no such version exists)
|
|
await finder.findPythonVersion('3.x', 'x64');
|
|
});
|
|
|
|
it('Finds Python if it is not installed, but exists in the manifest', async () => {
|
|
const findSpy: jest.SpyInstance = jest.spyOn(
|
|
installer,
|
|
'findReleaseFromManifest'
|
|
);
|
|
findSpy.mockImplementation(() => <tc.IToolRelease>pythonRelease);
|
|
|
|
const installSpy: jest.SpyInstance = jest.spyOn(
|
|
installer,
|
|
'installCpythonFromRelease'
|
|
);
|
|
installSpy.mockImplementation(async () => {
|
|
const pythonDir: string = path.join(toolDir, 'Python', '1.2.3', 'x64');
|
|
await io.mkdirP(pythonDir);
|
|
fs.writeFileSync(`${pythonDir}.complete`, 'hello');
|
|
});
|
|
// This will throw if it doesn't find it in the cache and in the manifest (because no such version exists)
|
|
await finder.findPythonVersion('1.2.3', 'x64');
|
|
});
|
|
|
|
it('Errors if Python is not installed', async () => {
|
|
// This will throw if it doesn't find it in the cache and in the manifest (because no such version exists)
|
|
let thrown = false;
|
|
try {
|
|
await finder.findPythonVersion('3.300000', 'x64');
|
|
} catch {
|
|
thrown = true;
|
|
}
|
|
expect(thrown).toBeTruthy();
|
|
});
|
|
|
|
it('Finds PyPy if it is installed', async () => {
|
|
const pythonDir: string = path.join(toolDir, 'PyPy', '2.0.0', 'x64');
|
|
await io.mkdirP(pythonDir);
|
|
fs.writeFileSync(`${pythonDir}.complete`, 'hello');
|
|
// This will throw if it doesn't find it in the cache (because no such version exists)
|
|
await finder.findPythonVersion('pypy2', 'x64');
|
|
});
|
|
});
|